Quantities example¶
This example shows quantities functionality.
The code¶
import quantities as pq
from pylatex import Document, Math, Quantity, Section, Subsection
if __name__ == "__main__":
doc = Document()
section = Section("Quantity tests")
subsection = Subsection("Scalars with units")
G = pq.constants.Newtonian_constant_of_gravitation
moon_earth_distance = 384400 * pq.km
moon_mass = 7.34767309e22 * pq.kg
earth_mass = 5.972e24 * pq.kg
moon_earth_force = G * moon_mass * earth_mass / moon_earth_distance**2
q1 = Quantity(
moon_earth_force.rescale(pq.newton),
options={"round-precision": 4, "round-mode": "figures"},
)
math = Math(data=["F=", q1])
subsection.append(math)
section.append(subsection)
subsection = Subsection("Scalars without units")
world_population = 7400219037
N = Quantity(
world_population,
options={"round-precision": 2, "round-mode": "figures"},
format_cb="{0:23.17e}".format,
)
subsection.append(Math(data=["N=", N]))
section.append(subsection)
subsection = Subsection("Scalars with uncertainties")
width = pq.UncertainQuantity(7.0, pq.meter, 0.4)
length = pq.UncertainQuantity(6.0, pq.meter, 0.3)
area = Quantity(
width * length,
options="separate-uncertainty",
format_cb=lambda x: "{0:.1f}".format(float(x)),
)
subsection.append(Math(data=["A=", area]))
section.append(subsection)
doc.append(section)
doc.generate_pdf("quantities_ex", clean_tex=False)
The generated files¶
quantities_ex.tex¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | \documentclass{article}% \usepackage[T1]{fontenc}% \usepackage[utf8]{inputenc}% \usepackage{lmodern}% \usepackage{textcomp}% \usepackage{lastpage}% \usepackage{amsmath}% \usepackage[separate-uncertainty=true]{siunitx}% \DeclareSIUnit\rpm{rpm}% % % % \begin{document}% \normalsize% \section{Quantity tests}% \label{sec:Quantitytests}% \subsection{Scalars with units}% \label{subsec:Scalarswithunits}% \[% F= \SI[round-precision=4,round-mode=figures]{1.9820166063855052e+20}{\newton}% \] % \subsection{Scalars without units}% \label{subsec:Scalarswithoutunits}% \[% N= \num[round-precision=2,round-mode=figures]{7.40021903700000000e+09}% \] % \subsection{Scalars with uncertainties}% \label{subsec:Scalarswithuncertainties}% \[% A= \SI[separate-uncertainty]{42.0 +- 3.2}{\meter\tothe{2}}% \] % \end{document} |
quantities_ex.pdf
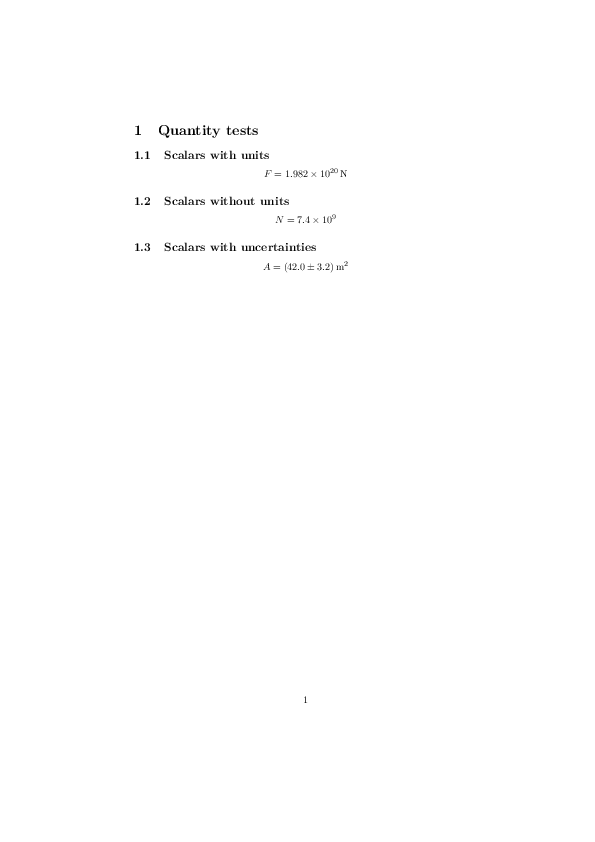