Basic inheritance example¶
This example shows basic document generation functionality by inheritance.
The code¶
from pylatex import Command, Document, Section, Subsection
from pylatex.utils import NoEscape, italic
class MyDocument(Document):
def __init__(self):
super().__init__()
self.preamble.append(Command("title", "Awesome Title"))
self.preamble.append(Command("author", "Anonymous author"))
self.preamble.append(Command("date", NoEscape(r"\today")))
self.append(NoEscape(r"\maketitle"))
def fill_document(self):
"""Add a section, a subsection and some text to the document."""
with self.create(Section("A section")):
self.append("Some regular text and some ")
self.append(italic("italic text. "))
with self.create(Subsection("A subsection")):
self.append("Also some crazy characters: $&#{}")
if __name__ == "__main__":
# Document
doc = MyDocument()
# Call function to add text
doc.fill_document()
# Add stuff to the document
with doc.create(Section("A second section")):
doc.append("Some text.")
doc.generate_pdf("basic_inheritance", clean_tex=False)
tex = doc.dumps() # The document as string in LaTeX syntax
The generated files¶
basic_inheritance.tex¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | \documentclass{article}% \usepackage[T1]{fontenc}% \usepackage[utf8]{inputenc}% \usepackage{lmodern}% \usepackage{textcomp}% \usepackage{lastpage}% % \title{Awesome Title}% \author{Anonymous author}% \date{\today}% % \begin{document}% \normalsize% \maketitle% \section{A section}% \label{sec:Asection}% Some regular text and some % \textit{italic text. }% \subsection{A subsection}% \label{subsec:Asubsection}% Also some crazy characters: \$\&\#\{\} % \section{A second section}% \label{sec:Asecondsection}% Some text. % \end{document} |
basic_inheritance.pdf
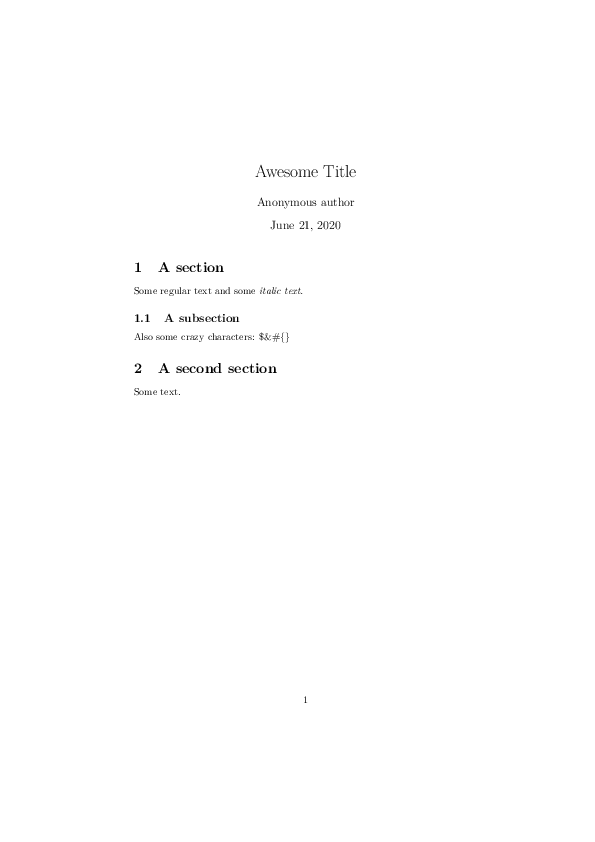