Tikzdraw example¶
This example shows TikZ drawing capabilities.
The code¶
from pylatex import (
Document,
TikZ,
TikZCoordinate,
TikZDraw,
TikZNode,
TikZOptions,
TikZUserPath,
)
if __name__ == "__main__":
# create document
doc = Document()
# add our sample drawings
with doc.create(TikZ()) as pic:
# options for our node
node_kwargs = {"align": "center", "minimum size": "100pt", "fill": "black!20"}
# create our test node
box = TikZNode(
text="My block",
handle="box",
at=TikZCoordinate(0, 0),
options=TikZOptions("draw", "rounded corners", **node_kwargs),
)
# add to tikzpicture
pic.append(box)
# draw a few paths
pic.append(
TikZDraw(
[TikZCoordinate(0, -6), "rectangle", TikZCoordinate(2, -8)],
options=TikZOptions(fill="red"),
)
)
# show use of anchor, relative coordinate
pic.append(TikZDraw([box.west, "--", "++(-1,0)"]))
# demonstrate the use of the with syntax
with pic.create(TikZDraw()) as path:
# start at an anchor of the node
path.append(box.east)
# necessary here because 'in' is a python keyword
path_options = {"in": 90, "out": 0}
path.append(TikZUserPath("edge", TikZOptions("-latex", **path_options)))
path.append(TikZCoordinate(1, 0, relative=True))
doc.generate_pdf("tikzdraw", clean_tex=False)
The generated files¶
tikzdraw.tex¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | \documentclass{article}% \usepackage[T1]{fontenc}% \usepackage[utf8]{inputenc}% \usepackage{lmodern}% \usepackage{textcomp}% \usepackage{lastpage}% \usepackage{tikz}% % % % \begin{document}% \normalsize% \begin{tikzpicture}% \node[draw,rounded corners,align=center,minimum size=100pt,fill=black!20] (box) at (0.0,0.0) {My block};% \path[draw,fill=red] (0.0,-6.0) rectangle (2.0,-8.0);% \path[draw] (box.west) -- ++(-1.0,0.0);% \path[draw] (box.east) edge[-latex,in=90,out=0] ++(1.0,0.0);% \end{tikzpicture}% \end{document} |
tikzdraw.pdf
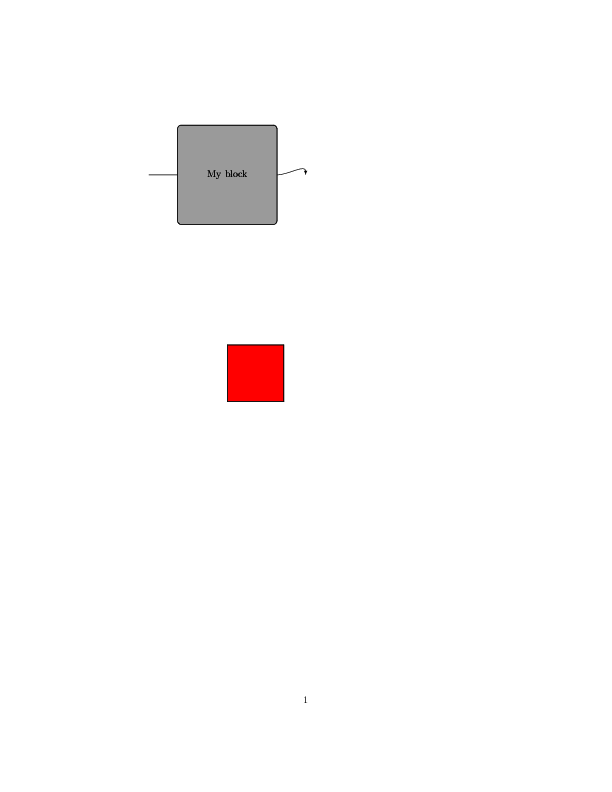