Numpy example¶
This example shows numpy functionality.
The code¶
import numpy as np
from pylatex import Document, Math, Matrix, Section, Subsection, VectorName
if __name__ == "__main__":
a = np.array([[100, 10, 20]]).T
doc = Document()
section = Section("Numpy tests")
subsection = Subsection("Array")
vec = Matrix(a)
vec_name = VectorName("a")
math = Math(data=[vec_name, "=", vec])
subsection.append(math)
section.append(subsection)
subsection = Subsection("Matrix")
M = np.matrix([[2, 3, 4], [0, 0, 1], [0, 0, 2]])
matrix = Matrix(M, mtype="b")
math = Math(data=["M=", matrix])
subsection.append(math)
section.append(subsection)
subsection = Subsection("Product")
math = Math(data=["M", vec_name, "=", Matrix(M * a)])
subsection.append(math)
section.append(subsection)
doc.append(section)
doc.generate_pdf("numpy_ex", clean_tex=False)
The generated files¶
numpy_ex.tex¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 | \documentclass{article}% \usepackage[T1]{fontenc}% \usepackage[utf8]{inputenc}% \usepackage{lmodern}% \usepackage{textcomp}% \usepackage{lastpage}% \usepackage{amsmath}% % % % \begin{document}% \normalsize% \section{Numpy tests}% \label{sec:Numpytests}% \subsection{Array}% \label{subsec:Array}% \[% \mathbf{a} = \begin{pmatrix}% 100\\% 10\\% 20% \end{pmatrix}% \] % \subsection{Matrix}% \label{subsec:Matrix}% \[% M= \begin{bmatrix}% 2&3&4\\% 0&0&1\\% 0&0&2% \end{bmatrix}% \] % \subsection{Product}% \label{subsec:Product}% \[% M \mathbf{a} = \begin{pmatrix}% 310\\% 20\\% 40% \end{pmatrix}% \] % \end{document} |
numpy_ex.pdf
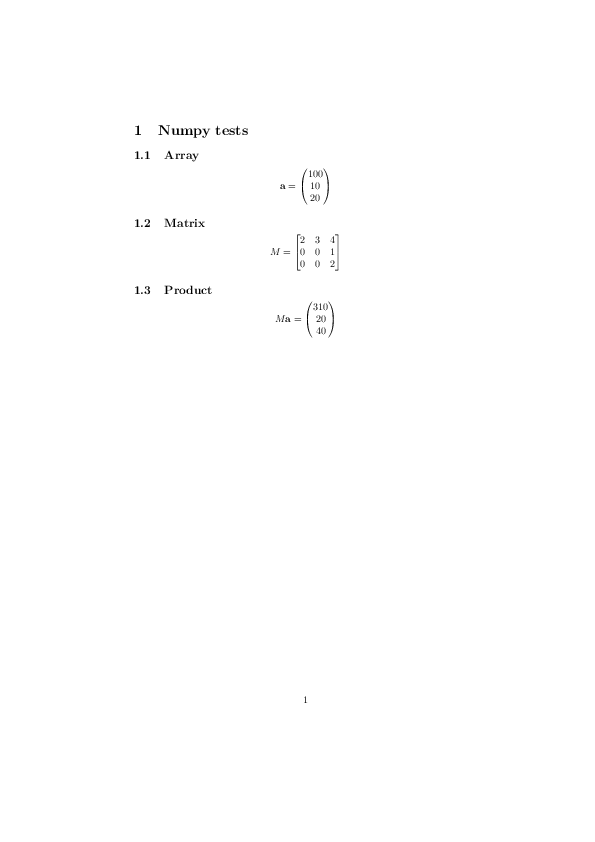