Environment example¶
Wrapping existing LaTeX environments with the Environment class.
-
class
AllTT
(*, options=None, arguments=None, start_arguments=None, **kwargs)[source]¶ A class to wrap LaTeX’s alltt environment.
Parameters:
The code¶
from pylatex import Document, Section
from pylatex.base_classes import Environment
from pylatex.package import Package
from pylatex.utils import NoEscape
class AllTT(Environment):
"""A class to wrap LaTeX's alltt environment."""
packages = [Package("alltt")]
escape = False
content_separator = "\n"
# Create a new document
doc = Document()
with doc.create(Section("Wrapping Latex Environments")):
doc.append(
NoEscape(
r"""
The following is a demonstration of a custom \LaTeX{}
command with a couple of parameters.
"""
)
)
# Put some data inside the AllTT environment
with doc.create(AllTT()):
verbatim = (
"This is verbatim, alltt, text.\n\n\n"
"Setting \\underline{escape} to \\underline{False} "
"ensures that text in the environment is not\n"
"subject to escaping...\n\n\n"
"Setting \\underline{content_separator} "
"ensures that line endings are broken in\n"
"the latex just as they are in the input text.\n"
"alltt supports math: \\(x^2=10\\)"
)
doc.append(verbatim)
doc.append("This is back to normal text...")
# Generate pdf
doc.generate_pdf("environment_ex", clean_tex=False)
The generated files¶
environment_ex.tex¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | \documentclass{article}% \usepackage[T1]{fontenc}% \usepackage[utf8]{inputenc}% \usepackage{lmodern}% \usepackage{textcomp}% \usepackage{lastpage}% \usepackage{alltt}% % % % \begin{document}% \normalsize% \section{Wrapping Latex Environments}% \label{sec:WrappingLatexEnvironments}% The following is a demonstration of a custom \LaTeX{} command with a couple of parameters. % \begin{alltt} This is verbatim, alltt, text. Setting \underline{escape} to \underline{False} ensures that text in the environment is not subject to escaping... Setting \underline{content_separator} ensures that line endings are broken in the latex just as they are in the input text. alltt supports math: \(x^2=10\) \end{alltt}% This is back to normal text... % \end{document} |
environment_ex.pdf
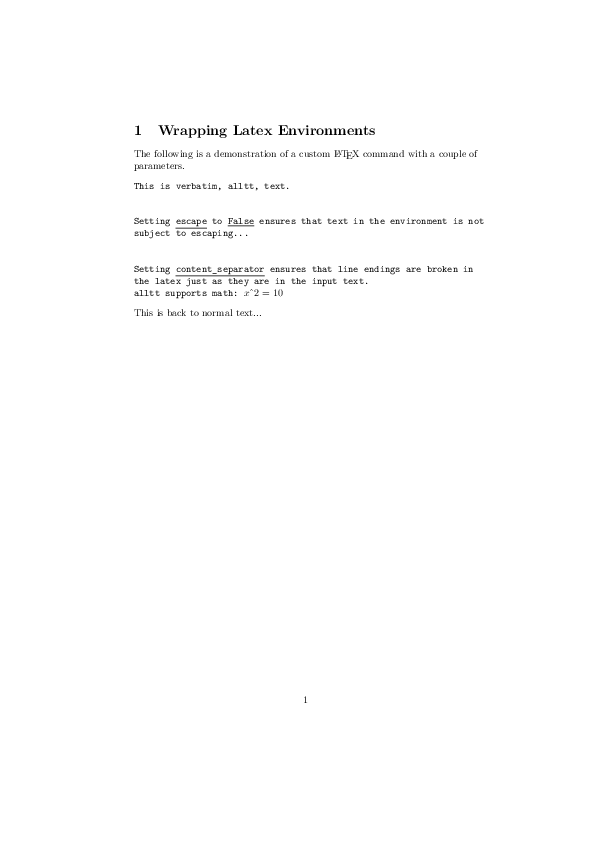