Own commands example¶
How to represent your own LaTeX commands and environments in PyLaTeX.
-
class
ExampleEnvironment
(*, options=None, arguments=None, start_arguments=None, **kwargs)[source]¶ A class representing a custom LaTeX environment.
This class represents a custom LaTeX environment named
exampleEnvironment
.Parameters:
-
class
ExampleCommand
(arguments=None, options=None, *, extra_arguments=None)[source]¶ A class representing a custom LaTeX command.
This class represents a custom LaTeX command named
exampleCommand
.Parameters: - arguments (None, str, list or
Arguments
) – The main arguments of the command. - options (None, str, list or
Options
) – Options of the command. These are placed in front of the arguments. - extra_arguments (None, str, list or
Arguments
) – Extra arguments for the command. When these are supplied the options will be placed before them instead of before the normal arguments. This allows for a way of having one or more arguments before the options.
- arguments (None, str, list or
The code¶
from pylatex import Document, Section, UnsafeCommand
from pylatex.base_classes import Arguments, CommandBase, Environment
from pylatex.package import Package
from pylatex.utils import NoEscape
class ExampleEnvironment(Environment):
"""
A class representing a custom LaTeX environment.
This class represents a custom LaTeX environment named
``exampleEnvironment``.
"""
_latex_name = "exampleEnvironment"
packages = [Package("mdframed")]
class ExampleCommand(CommandBase):
"""
A class representing a custom LaTeX command.
This class represents a custom LaTeX command named
``exampleCommand``.
"""
_latex_name = "exampleCommand"
packages = [Package("color")]
# Create a new document
doc = Document()
with doc.create(Section("Custom commands")):
doc.append(
NoEscape(
r"""
The following is a demonstration of a custom \LaTeX{}
command with a couple of parameters.
"""
)
)
# Define the new command
new_comm = UnsafeCommand(
"newcommand",
"\exampleCommand",
options=3,
extra_arguments=r"\color{#1} #2 #3 \color{black}",
)
doc.append(new_comm)
# Use our newly created command with different arguments
doc.append(ExampleCommand(arguments=Arguments("blue", "Hello", "World!")))
doc.append(ExampleCommand(arguments=Arguments("green", "Hello", "World!")))
doc.append(ExampleCommand(arguments=Arguments("red", "Hello", "World!")))
with doc.create(Section("Custom environments")):
doc.append(
NoEscape(
r"""
The following is a demonstration of a custom \LaTeX{}
environment using the mdframed package.
"""
)
)
# Define a style for our box
mdf_style_definition = UnsafeCommand(
"mdfdefinestyle",
arguments=[
"my_style",
("linecolor=#1," "linewidth=#2," "leftmargin=1cm," "leftmargin=1cm"),
],
)
# Define the new environment using the style definition above
new_env = UnsafeCommand(
"newenvironment",
"exampleEnvironment",
options=2,
extra_arguments=[
mdf_style_definition.dumps() + r"\begin{mdframed}[style=my_style]",
r"\end{mdframed}",
],
)
doc.append(new_env)
# Usage of the newly created environment
with doc.create(ExampleEnvironment(arguments=Arguments("red", 3))) as environment:
environment.append("This is the actual content")
# Generate pdf
doc.generate_pdf("own_commands_ex", clean_tex=False)
The generated files¶
own_commands_ex.tex¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | \documentclass{article}% \usepackage[T1]{fontenc}% \usepackage[utf8]{inputenc}% \usepackage{lmodern}% \usepackage{textcomp}% \usepackage{lastpage}% \usepackage{color}% \usepackage{mdframed}% % % % \begin{document}% \normalsize% \section{Custom commands}% \label{sec:Customcommands}% The following is a demonstration of a custom \LaTeX{} command with a couple of parameters. % \newcommand{\exampleCommand}[3]{\color{#1} #2 #3 \color{black}}% \exampleCommand{blue}{Hello}{World!}% \exampleCommand{green}{Hello}{World!}% \exampleCommand{red}{Hello}{World!} % \section{Custom environments}% \label{sec:Customenvironments}% The following is a demonstration of a custom \LaTeX{} environment using the mdframed package. % \newenvironment{exampleEnvironment}[2]{\mdfdefinestyle{my_style}{linecolor=#1,linewidth=#2,leftmargin=1cm,leftmargin=1cm}\begin{mdframed}[style=my_style]}{\end{mdframed}}% \begin{exampleEnvironment}{red}{3}% This is the actual content% \end{exampleEnvironment} % \end{document} |
own_commands_ex.pdf
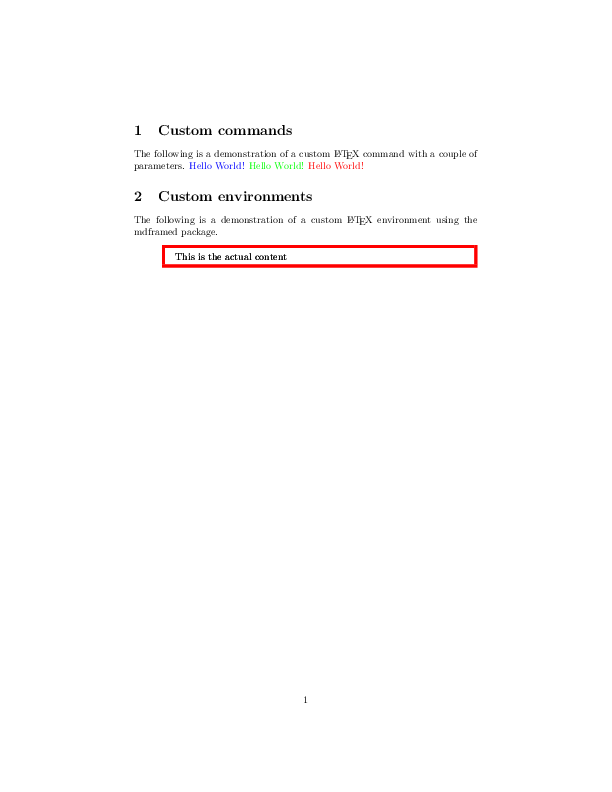